What is Space and Time Complexity in Data Structure
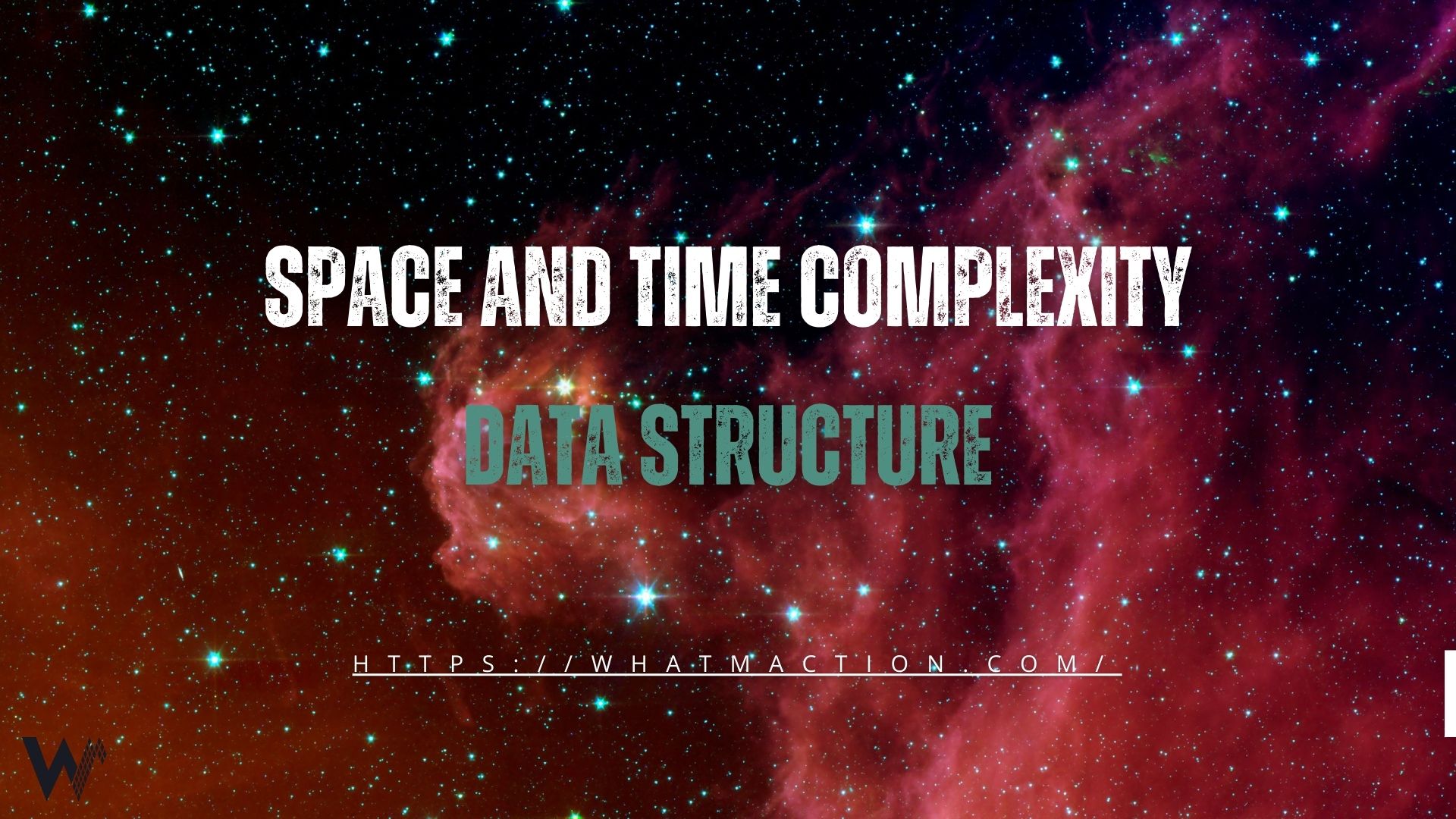
When you’re solving problems in computer science, especially when you’re preparing for coding interviews, it’s really important to understand the efficiency of your algorithms. There are two primary measures of efficiency: time complexity and space complexity. Both of these metrics help evaluate how well an algorithm performs, but in different ways. In this post, we will break down both concepts, how to calculate them, and why they matter.
What is Time Complexity?
Time complexity estimates how fast or slow an algorithm runs based on the input size.
Example :
• If you have a small list of numbers, sorting it takes very little time, but with a humongous list, it could take significantly longer.
Time complexity helps predict how the algorithm will perform when the input is large.
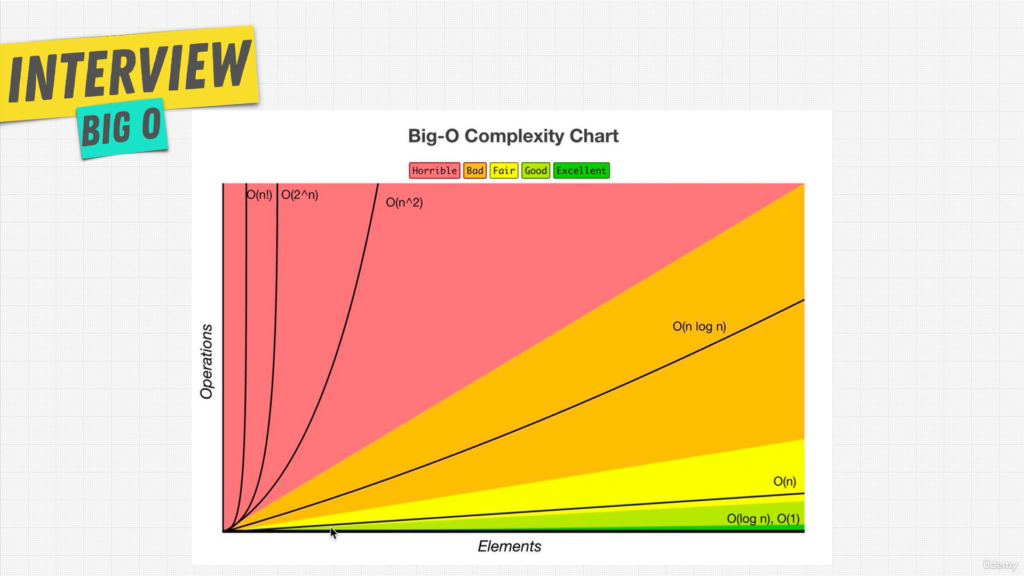
To Understand more and for the cheatSheet https://www.bigocheatsheet.com/
What is Big O Notation?
Big O notation is a way to describe the time complexity in the simplest and most general way. The best way to describe it is the worst-case scenario for how long an algorithm will take to run based on the size of the input.
For instance :
• An algorithm with a time complexity of O(n) means its time grows linearly with the input size; that is, when the input size doubles, the time roughly doubles.
• If an algorithm has a time complexity of O(n ²), it means that the time increases quadratically with the size of input. If the size of input doubles, then time might quadruple.
-> Common Time Complexities
- O(1) – Constant Time : The algorithm Execution time is Constant no matter of the Input size Data.
def constant_time_example(arr):
return arr[0] # O(1)
- O(n) – Linear Time : The Execution time Grows Linearly with size of the Input.
def find_element(arr, x):
for i in arr:
if i == x:
return True
return False
- O(n^2) – Quadratic Time : The Execution increases quadratically with the input size. This is true for algorithms containing nested loops.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
- O(log n) – Logarithmic Time : The Execution time increases logarithmic in the input size. This often occurs when the algorithm recursively halves the problem.
def logarithmic_time_example(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid # O(log n)
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
- O(n log n) – Log-Linear Time : This is the time complexity of many efficient sorting algorithms, like Merge Sort and Quick Sort.
def log_linear_time_example(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left_half = log_linear_time_example(arr[:mid])
right_half = log_linear_time_example(arr[mid:])
return merge(left_half, right_half) # O(n log n)
What is Space Complexity?
Space Complexity is defined as the amount of memory space required for an algorithm in terms of the size of its input. Just like time complexity measures runtime, space complexity measures the amount of memory that your algorithm needs.
Most often, the space complexity is expressed in Big O notation as well.
-> Some Common Space Complexities
- O(1) – Constant Space : The memory usage does not depend on the input size.
def constant_space_example(n):
count = 0 # O(1)
for i in range(n):
count += i
return count
- O(n) – Linear Space : The space required increases linearly with the input size.
def linear_space_example(n):
arr = [0] * n # O(n)
return arr
- O(n^2) – Quadratic Space : The space required increases quadratically with the input size. This happens when you use a 2D array or matrix.
def quadratic_space_example(n):
matrix = [[0] * n for _ in range(n)] # O(n^2)
return matrix
- O(log n) – Logarithmic Space : Space grows logarithmically. This is usually found in recursive algorithms such as binary search (if implemented recursively) or recursive tree traversals.
def logarithmic_space_example(n):
if n == 0:
return 0
return logarithmic_space_example(n // 2) + 1 # O(log n)
Why Time and Space Complexity Matter
Efficiency :
Understanding time and space complexity helps you optimize your algorithm. For instance, if an algorithm has a time complexity of O(n^2), it might work for small inputs, but for large datasets, it could become prohibitively slow. By identifying the complexity, you can decide whether a more efficient algorithm is needed.
Scalability:
As the size of your input grows larger, algorithms that are high in terms of time or space complexity can make your program run too slow or consume excessive memory. Better algorithms should scale well with large inputs.
Real-World Applications:
Optimization of both time and space complexities in real-world systems like databases, search engines, and networks will let them respond faster and use the resources more efficiently.
How to Calculate Time and Space Complexity?
- Time Complexity :
Count Operations : How many basic operations (comparisons, assignments, etc.) an algorithm takes based on the input size.
Identify Loops and Recursion : Loops and recursive calls usually determine the time complexity. Multiply the complexities for nested loops
Use Big O notation : Represent the highest-order growth; ignore constant factors and lower-order terms
- Space Complexity :
Consider Variables and Data Structures: Compute how much extra memory is being used compared to the input. For instance, an algorithm which uses some large array, has a space complexity directly proportional to the size of the array.
Consider Recursive Stack : When coming to the recursive algorithms, then the space complexity also includes the space taken up by call stack.
Time and space complexity are what it takes for anyone to become a good developer, and optimizing one’s code requires knowing what constitutes an optimal complexity in order to become an excellent problem-solver. For these reasons, input size always goes hand-in-hand with algorithms trying to minimize run time to get around any given large inputs, thereby keeping run-time problems to the lowest end.
The next time you meet a problem, take a little time to analyze its time and space complexity. And it will help you decide which algorithm is best suited for the task at hand.
Happy coding!