Document Object Model (DOM) in JavaScript 2025
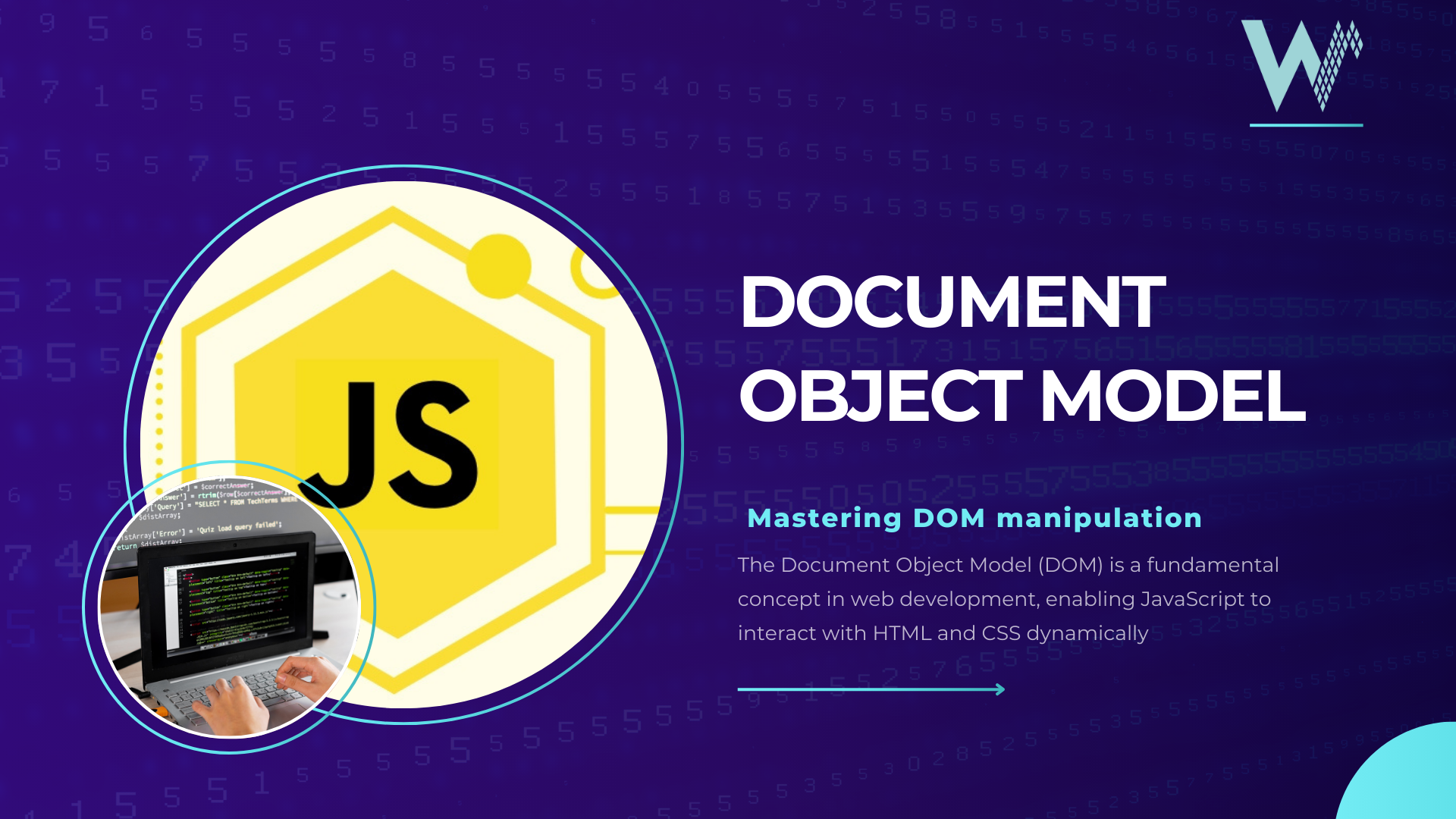
Introduction
The Document Object Model (DOM) is a fundamental concept in web development, enabling JavaScript to interact with HTML and CSS dynamically. Mastering Document Object Model manipulation allows developers to create highly interactive, efficient, and engaging web applications.
This article provides an in-depth exploration of JavaScript Document Object Model, covering its structure, methods for accessing and modifying elements, event handling, best practices, and performance optimization techniques.
What is the Document Object Model (DOM)?
The DOM represents an HTML document as a structured tree of elements, which JavaScript can access and manipulate.
Key Features of the DOM:
- Tree-Like Structure: HTML is represented hierarchically.
- Dynamic Content Manipulation: JavaScript enables real-time changes.
- Event Handling: React to user interactions efficiently.
- Cross-Browser Compatibility: Ensures consistency across all browsers.
Example of an HTML Document and its DOM Representation
<!DOCTYPE html>
<html>
<head>
<title>DOM Example</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<h1>Hello, World!</h1>
<h2>This is heading 2 </h2>
</body>
</html>
The corresponding DOM structure:

Accessing Elements in the DOM
JavaScript provides several methods to select HTML elements:
1. getElementById()
Selects an element by its id.
let heading = document.getElementById("title");
console.log(heading.innerText); // Output: Hello, World!
2. getElementsByClassName()
Retrieves all elements with a given class name.
let paragraphs = document.getElementsByClassName("text");
console.log(paragraphs[0].innerText);
3. getElementsByTagName()
Selects elements based on their tag name
let allParagraphs = document.getElementsByTagName("p");
console.log(allParagraphs.length);
4. querySelector()
and querySelectorAll()
Uses CSS selectors to select elements.
let firstParagraph = document.querySelector("p");
let allItems = document.querySelectorAll(".text");
Modifying the DOM
JavaScript allows modifying content, attributes, and styles dynamically.
1. Changing Inner Content
document.getElementById("title").innerText = "Welcome to JavaScript DOM!";
document.getElementById("title").innerHTML = "<strong>Welcome!</strong>";
2. Modifying Attributes
document.querySelector("img").setAttribute("src", "image.jpg");
3. Changing Styles Dynamically
document.querySelector("h1").style.color = "blue";
document.querySelector("h1").style.fontSize = "30px";
Adding and Removing Elements
1. Creating New Elements
let newElement = document.createElement("p");
newElement.innerText = "This is a dynamically added paragraph.";
document.body.appendChild(newElement);
2. Removing Elements
let paragraph = document.querySelector(".text");
paragraph.remove();
Event Handling in the DOM
Handling events is essential for interactive web applications.
1. Adding Event Listeners
document.getElementById("title").addEventListener("click", function() {
alert("Title clicked!");
});
2. Using Event Object
document.getElementById("title").addEventListener("mouseover", function(event) {
event.target.style.color = "red";
});
Best Practices for Optimizing DOM Manipulation
1. Minimize Repaints and Reflows
- Modify elements outside the DOM tree before appending.
- Use
documentFragment
to group changes before adding them to the document.
2. Use Event Delegation
- Instead of adding event listeners to multiple child elements, use a single listener at the parent level.
document.querySelector("ul").addEventListener("click", function(event) {
if (event.target.tagName === "LI") {
console.log("List item clicked:", event.target.innerText);
}
});
3. Optimize Selectors
- Use
querySelectorAll()
efficiently by targeting specific elements instead of broad queries.
4. Avoid Unnecessary DOM Access
- Store references to elements instead of repeatedly selecting them.
let button = document.getElementById("btn");
button.addEventListener("click", function() {
console.log("Button Clicked");
});
5. How can I improve DOM performance?
To optimize performance, minimize repaints and reflows, use event delegation, store element references, and batch DOM updates using documentFragment
.
Additionally, avoid inline styles and excessive DOM manipulations within loops. Use CSS classes instead of modifying styles directly in JavaScript
Conclusion
Understanding the Document Object Model (DOM) is essential for JavaScript developers. Mastering DOM manipulation, event handling, and optimization techniques allows you to create efficient and interactive web applications.
By applying best practices, you can enhance website performance and provide a seamless user experience.
Start experimenting with JavaScript DOM Manipulation today and take your web development skills to the next level! Visit Whatmaction.com for more insights.
For further reading on programming concepts, check out this detailed guide on Object-Oriented Programming (OOP).