Advanced Asynchronous Programming in Python with Asyncio: A Deep Dive into High-Performance Concurrency
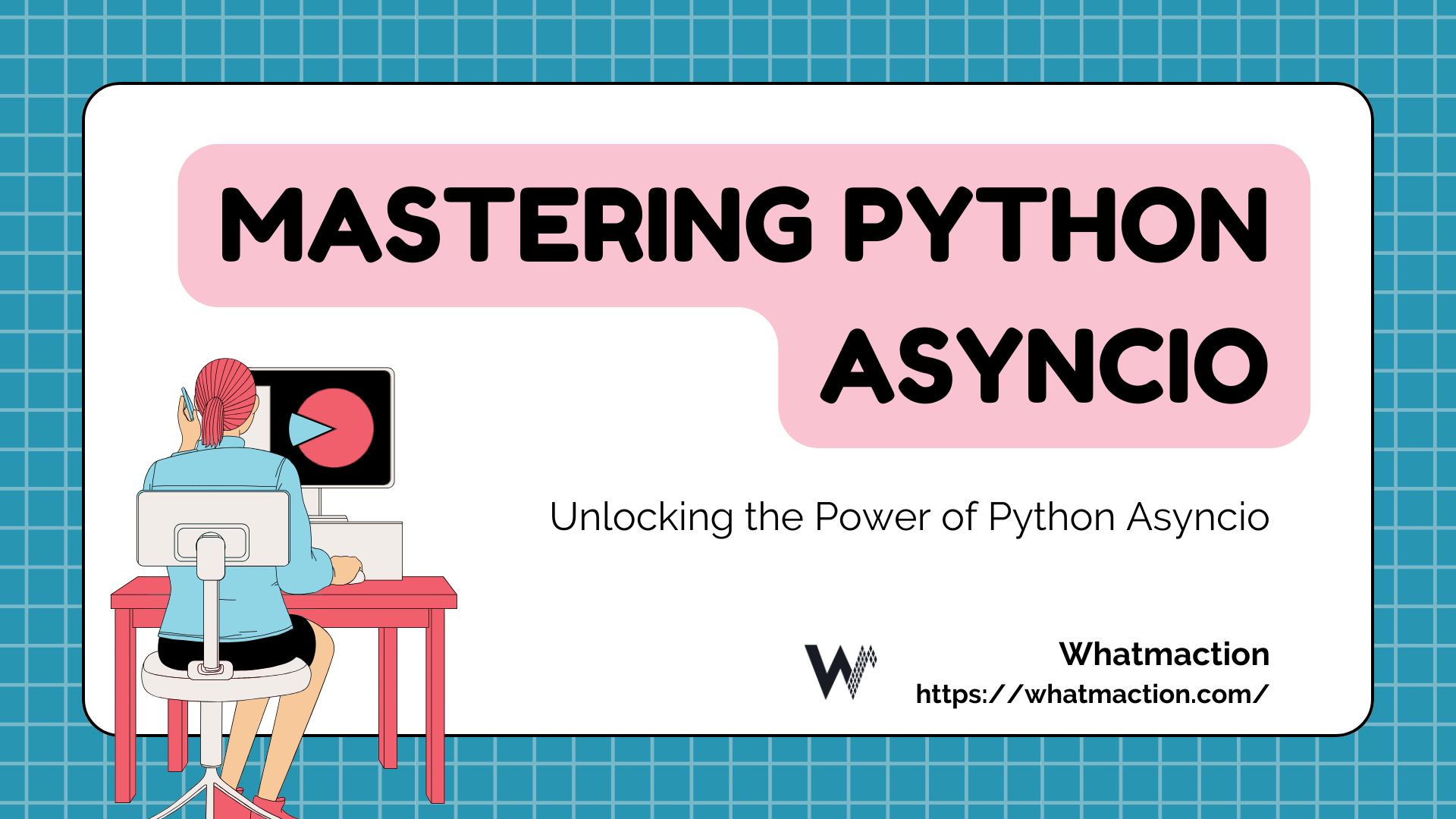
In today’s world of rapidly scaling web applications, network servers, and real-time data processing systems, achieving high concurrency and efficiency in Python is more critical than ever. Traditional multi-threading and multi-processing solutions can sometimes fall short due to Python’s Global Interpreter Lock (GIL) and the overhead associated with context switching. This is where asynchronous programming shines, enabling developers to write highly concurrent and efficient applications without the burden of heavy threads or processes.
In this comprehensive guide, we will dive deep into advanced asynchronous programming in Python using the Asyncio library. We’ll cover its core concepts, explore how it leverages event loops, coroutines, and tasks, and examine best practices and patterns to harness its full potential. Whether you’re building a high-performance web server, an I/O-bound application, or a real-time data processor, mastering Asyncio can be a game-changer in your development toolkit.
Introduction to Asynchronous Programming in Python
Asynchronous programming is a paradigm that allows for the execution of tasks concurrently, without waiting for each task to complete before moving on to the next. In Python, this is achieved primarily through the Asyncio library, which uses an event loop to manage the execution of asynchronous code. Instead of blocking the execution while waiting for I/O operations (like network requests or file I/O), asynchronous programming lets the program continue executing other tasks. This approach can lead to dramatic improvements in performance and responsiveness, particularly in I/O-bound applications.
Traditional methods such as multi-threading and multi-processing can be used to achieve concurrency, but they come with their own set of challenges. Threads, for example, can lead to race conditions and are often hampered by the Global Interpreter Lock (GIL) in CPython, which prevents true parallel execution of Python bytecodes. In contrast, Asyncio uses cooperative multitasking, where tasks voluntarily yield control, allowing a single thread to manage many concurrent operations efficiently.
Core Concepts of Asyncio
Event Loop
At the heart of Asyncio is the event loop. The event loop is responsible for scheduling and managing asynchronous tasks. It continuously monitors and dispatches events, such as I/O operations, ensuring that tasks are executed when they are ready. The event loop is central to the asynchronous programming model in Python, and understanding its workings is key to leveraging Asyncio effectively.
When you write an asynchronous function (a coroutine) in Python, the event loop manages its execution. The loop checks for coroutines that are ready to run and executes them until they yield control (often because they are waiting on an I/O operation). Once the awaited I/O is complete, the event loop resumes the coroutine, continuing its execution.
Coroutines
Coroutines are the building blocks of asynchronous programming in Python. Defined with the async def
syntax, coroutines are functions that can pause execution using the await
keyword, allowing other tasks to run concurrently. This non-blocking behavior makes coroutines ideal for I/O-bound operations where waiting for an external process (like a network call) would normally halt program execution.
Here’s a simple example of a coroutine:
import asyncio
async def fetch_data(url):
print(f"Fetching data from {url}")
await asyncio.sleep(2) # Simulate an I/O-bound task
print(f"Finished fetching data from {url}")
return f"Data from {url}"
# Running the coroutine using the event loop
async def main():
result = await fetch_data("https://example.com")
print(result)
asyncio.run(main())
In this example, fetch_data
is a coroutine that simulates an I/O operation using asyncio.sleep()
. The await
keyword pauses the coroutine, allowing the event loop to run other tasks during the sleep period.
Tasks and Future Objects
Tasks in Asyncio are wrappers for coroutines. When you schedule a coroutine to run, Asyncio wraps it in a Task object, which is then managed by the event loop. Tasks provide a way to track the execution of a coroutine, check its status, and retrieve its result once completed.
Future objects are similar in that they represent a value that will be available at some point in the future. While Tasks are a subclass of Future, they specifically represent the execution of a coroutine. Both Tasks and Future objects are integral to managing asynchronous workflows, as they enable you to coordinate multiple concurrent operations.
Concurrency with Asyncio
One of the primary benefits of Asyncio is its ability to handle multiple concurrent tasks efficiently. By using functions like asyncio.gather()
, developers can run several coroutines concurrently and wait for all of them to complete. This is particularly useful when you have multiple independent I/O-bound operations that can be performed in parallel.
For example:
async def main():
urls = ["https://example.com/1", "https://example.com/2", "https://example.com/3"]
tasks = [fetch_data(url) for url in urls]
results = await asyncio.gather(*tasks)
for result in results:
print(result)
asyncio.run(main())
In this snippet, three fetch operations are run concurrently, significantly reducing the total execution time compared to running them sequentially.
Advanced Patterns in Asynchronous Programming
Handling Exceptions in Asynchronous Code
Exception handling in asynchronous programming can be tricky due to the concurrent nature of tasks. It is important to wrap your asynchronous calls in try/except blocks to catch and handle exceptions gracefully. When using functions like asyncio.gather()
, you can specify return_exceptions=True
to ensure that all exceptions are returned as part of the results, rather than causing the entire process to fail.
Example:
async def fetch_with_error(url):
try:
# Simulating an error for demonstration
if "error" in url:
raise ValueError("An error occurred!")
await asyncio.sleep(1)
return f"Data from {url}"
except Exception as e:
return f"Error fetching {url}: {e}"
async def main():
urls = ["https://example.com/1", "https://example.com/error", "https://example.com/3"]
results = await asyncio.gather(*(fetch_with_error(url) for url in urls), return_exceptions=True)
for result in results:
print(result)
asyncio.run(main())
This approach ensures that even if one task fails, the overall process continues and you can handle errors on a per-task basis.
Using Semaphores to Limit Concurrency
In some cases, you may need to limit the number of concurrent operations, particularly when dealing with external services that impose rate limits. Asyncio provides semaphores to control access to a resource, allowing you to limit the number of concurrent coroutines.
For example:
async def limited_fetch(sem, url):
async with sem:
return await fetch_data(url)
async def main():
sem = asyncio.Semaphore(2) # Limit concurrency to 2 tasks
urls = ["https://example.com/1", "https://example.com/2", "https://example.com/3", "https://example.com/4"]
tasks = [limited_fetch(sem, url) for url in urls]
results = await asyncio.gather(*tasks)
for result in results:
print(result)
asyncio.run(main())
This ensures that only two fetch operations run concurrently, helping to prevent overloading the target service or exceeding rate limits.
Integrating Asyncio with Other Libraries
Asyncio can be integrated with various other Python libraries and frameworks to build powerful applications. For instance, you can use it with web frameworks like Aiohttp or FastAPI to build asynchronous web applications. Additionally, libraries like SQLAlchemy support asynchronous database operations, allowing you to write non-blocking code for data-intensive applications.
When integrating Asyncio with other libraries, it is essential to ensure that the external library supports asynchronous operations, or you may need to run blocking code in a separate thread or process using functions like asyncio.to_thread()
.
Advanced Debugging and Profiling Techniques
Debugging asynchronous code can be challenging due to the non-linear flow of execution. Asyncio provides tools for debugging, such as enabling debug mode in the event loop:
asyncio.get_event_loop().set_debug(True)
This mode provides more detailed logs and warnings about potential issues such as slow callbacks and resource leaks.
For profiling, you can use libraries like Py-Spy or the built-in cProfile
module, although special care must be taken when profiling asynchronous code. Profiling helps identify bottlenecks in your asynchronous workflows and provides insights into optimizing your code for better performance.
Best Practices for Writing Asynchronous Python Code
When developing asynchronous applications in Python, several best practices can help you write clean, efficient, and maintainable code:
- Avoid Blocking Calls:
Ensure that you do not use blocking functions in your coroutines. If you must use a blocking operation, consider running it in a separate thread usingasyncio.to_thread()
. - Leverage High-Level APIs:
Use Asyncio’s high-level APIs such asgather()
,wait()
, andas_completed()
to manage multiple concurrent tasks efficiently. These abstractions simplify the management of concurrent operations and make your code more readable. - Structure Your Code for Readability:
Keep your asynchronous code modular. Separate the logic for data fetching, processing, and error handling into distinct functions or classes. This modularity makes it easier to test and maintain your code. - Implement Robust Error Handling:
As previously discussed, always wrap your asynchronous calls in try/except blocks and use features likereturn_exceptions=True
when gathering tasks. This ensures that individual failures do not cascade and bring down your entire application. - Use Semaphores for Resource Management:
When interacting with external services or resources that have concurrency limits, use semaphores to control the number of concurrent accesses. This prevents resource exhaustion and ensures smoother operation. - Regularly Monitor and Log Activity:
Implement logging within your asynchronous code to monitor the behavior of your application. This is critical for debugging issues and understanding performance patterns in a live environment. - Stay Updated with Asyncio Developments:
Asyncio is an evolving library, and new features or improvements are regularly introduced. Stay informed about the latest updates and best practices from the Python community to ensure that your applications leverage the full potential of asynchronous programming.
Real-World Applications and Use Cases
To illustrate the power and versatility of Asyncio, let’s explore a few real-world use cases where asynchronous programming has made a significant impact.
Real-Time Data Processing
Many applications today require real-time data processing, such as streaming analytics, financial data processing, and IoT device management. Using Asyncio, developers can create pipelines that ingest, process, and analyze data streams concurrently without delay. This is crucial in environments where even a millisecond of delay can have significant consequences.
Asynchronous Web Servers
Modern web frameworks like FastAPI and Aiohttp leverage Asyncio to build high-performance web servers that handle thousands of simultaneous connections. By using non-blocking I/O operations, these servers can manage high traffic volumes with minimal latency, ensuring that web applications remain responsive even under heavy load.
Network Applications and Chatbots
Asyncio is ideal for network applications, including chat servers, messaging platforms, and real-time communication tools. The ability to handle many simultaneous connections efficiently makes it a natural fit for these types of applications. Chatbots, for instance, can use Asyncio to handle multiple user interactions concurrently, improving responsiveness and scalability.
Web Scraping and Data Aggregation
As discussed earlier, Asyncio can significantly speed up web scraping tasks by running multiple requests concurrently. This is particularly useful for aggregating data from various sources in real-time, enabling businesses to make data-driven decisions quickly. Combining Asyncio with libraries like BeautifulSoup or Aiohttp allows you to build robust, high-performance scraping solutions.
Advanced Asyncio Patterns and Techniques
Beyond the basic usage of coroutines and event loops, there are several advanced patterns and techniques that can further enhance the power of asynchronous programming in Python.
Chaining Coroutines and Task Dependencies
In complex applications, you might have multiple coroutines that depend on the output of previous ones. Chaining coroutines effectively can help manage these dependencies without blocking the event loop. This involves carefully structuring your coroutines so that data flows smoothly between tasks, often using constructs like await
within loops or nested coroutine calls.
Combining Asyncio with Synchronous Code
Sometimes, you may need to integrate asynchronous code with existing synchronous codebases. Python provides utilities like asyncio.run_coroutine_threadsafe()
and asyncio.to_thread()
to help bridge the gap between asynchronous and synchronous code. These tools allow you to offload blocking operations to separate threads, ensuring that the asynchronous workflow remains responsive.
Customizing the Event Loop
For highly specialized applications, you may need to customize the behavior of the event loop. Python’s Asyncio module allows you to create custom event loops or modify existing ones to better suit your application’s requirements. Custom event loops can be particularly useful in high-performance computing scenarios where fine-tuned scheduling can lead to significant performance gains.
Using Third-Party Async Libraries
There is a growing ecosystem of third-party libraries designed to work seamlessly with Asyncio. Libraries such as aiohttp
for web requests, aiomysql
for asynchronous MySQL database interactions, and aioredis
for Redis integration can greatly simplify the process of building asynchronous applications. Leveraging these libraries allows you to build full-featured, non-blocking applications with minimal boilerplate code.
Testing and Debugging Asynchronous Code
Testing asynchronous code can be more challenging than testing synchronous code due to its concurrent nature. However, there are several tools and techniques available to make this process easier:
- Async Testing Frameworks:
Frameworks likepytest-asyncio
extend pytest to support asynchronous tests, allowing you to write tests for your coroutines as if they were synchronous functions. - Logging and Debugging:
Enable Asyncio’s debug mode to get detailed insights into your event loop’s behavior. This mode can help you identify slow operations, unawaited coroutines, and other potential issues. - Mocking and Patching:
Use libraries likeunittest.mock
to simulate I/O operations and external API responses. This allows you to test your asynchronous code without relying on actual network calls or external services. - Profiling Tools:
Profiling asynchronous applications can be done using tools like Py-Spy or built-in modules such as cProfile. These tools help identify performance bottlenecks and areas where optimization is needed.
Conclusion
Advanced asynchronous programming in Python with Asyncio represents a paradigm shift in how developers build high-performance, concurrent applications. By leveraging the power of event loops, coroutines, and tasks, Asyncio enables you to handle I/O-bound operations with ease, significantly improving the responsiveness and scalability of your applications. From building real-time web servers and data processing pipelines to integrating with third-party services and APIs, the techniques discussed in this guide can be applied across a wide range of use cases.
Embracing asynchronous programming requires a shift in mindset—from thinking about sequential execution to managing concurrency in a cooperative, non-blocking manner. With robust error handling, proper resource management, and adherence to best practices, you can harness the full potential of Asyncio to build modern, efficient, and scalable Python applications.
As you continue to explore and implement advanced asynchronous programming techniques, remember that continuous learning and experimentation are key. Stay updated with the latest developments in Asyncio and the broader Python ecosystem, engage with the community, and continually refine your code to achieve optimal performance.
Whether you are building a new application from scratch or modernizing an existing codebase, advanced asynchronous programming with Asyncio offers a powerful toolset to overcome the limitations of traditional concurrency models. By investing the time to master these techniques, you can significantly enhance your applications’ performance, reduce latency, and create a smoother user experience—paving the way for innovation in the ever-evolving world of high-performance computing.
Written by an AI tool; minor mistakes may be present.